Class DetectFiducialSquareBinary<T extends ImageGray<T>>
- All Implemented Interfaces:
VerbosePrint
Square fiducial that encodes numerical values in a binary N by N grids, where N ≥ 3. The outer border is entirely black while the inner portion is divided into a grid of equally sized back and white squares. Typical grid sizes are 3x3, 4x4, and 5x5, which can encode up to 32, 4096, and 2,097,152 unique values respectively. In other words, a grid of size N can encode N*N-4 bits, or a number with 2N*N-4 values. The lower left corner is always back and while all the other corners are always white. This allows orientation to be uniquely determined.
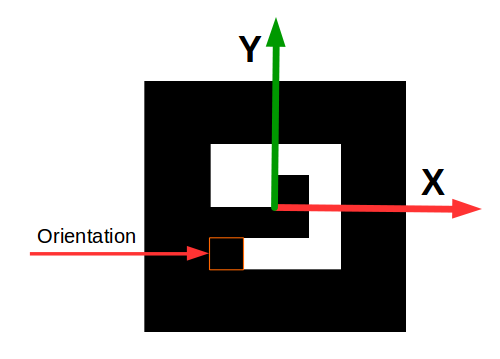
The above image is an example of a 4x4 grid and visually shows the fiducials internal coordinate system. The center of the fiducial is the origin of the coordinate system, e.g. all sides are width/2 distance away from the origin. +x is to the right, +y is up, and +z out of the paper towards the viewer. The black orientation corner is pointed out in the above image. The fiducial's width refers to the width of each side along the black border NOT the internal encoded image. The size of each square is the same and has a width of (fiducal width)*(1.0 - 2.0*(border fractional width))/N.
NOTE: While a larger grid size will allow you to encode more numbers it will increase the rate at which ID numbers
are incorrectly identified.
NOTE: The size of the border can be adjusted, but 0.25 is recommended. The thinner the black border is the worse
it will perform when viewed at an angle. However, the closer the fiducial is the less this is an issue allowing
for thinner borders.
-
Nested Class Summary
Nested classes/interfaces inherited from class boofcv.alg.fiducial.square.BaseDetectFiducialSquare
BaseDetectFiducialSquare.Result
-
Field Summary
FieldsFields inherited from class boofcv.alg.fiducial.square.BaseDetectFiducialSquare
borderWidthFraction
-
Constructor Summary
ConstructorsConstructorDescriptionDetectFiducialSquareBinary
(int gridWidth, double borderWidthFraction, double minimumBlackBorderFraction, InputToBinary<T> inputToBinary, DetectPolygonBinaryGrayRefine<T> quadDetector, Class<T> inputType) Configures the fiducial detector -
Method Summary
Modifier and TypeMethodDescriptionprotected int
Extract the numerical value it encodesprotected void
findBitCounts
(GrayF32 gray, double threshold) Converts the gray scale image into a binary number.int
Number of elements wide the grid islong
void
protected boolean
processSquare
(GrayF32 gray, BaseDetectFiducialSquare.Result result, double edgeInside, double edgeOutside) Processes the detected square and matches it to a known fiducial.protected void
void
setAmbiguityThreshold
(double ambiguityThreshold) parameters which specifies how tolerant it is of a square being ambiguous black or white.void
setLengthSide
(double lengthSide) protected boolean
Sees how many pixels were positive and negative in each square region.Methods inherited from class boofcv.alg.fiducial.square.BaseDetectFiducialSquare
computeFractionBoundary, configure, getBinary, getFound, process, setVerbose
-
Field Details
-
w
protected static final int w- See Also:
-
N
protected static final int N- See Also:
-
-
Constructor Details
-
DetectFiducialSquareBinary
public DetectFiducialSquareBinary(int gridWidth, double borderWidthFraction, double minimumBlackBorderFraction, InputToBinary<T> inputToBinary, DetectPolygonBinaryGrayRefine<T> quadDetector, Class<T> inputType) Configures the fiducial detector- Parameters:
gridWidth
- Number of elements wide the encoded square grid is. 3,4, or 5 is recommended.borderWidthFraction
- Fraction of the fiducial's width that the border occupies. 0.25 is recommended.inputToBinary
- Converts the input image into a binary imagequadDetector
- Detects quadrilaterals in the input imageinputType
- Type of image it's processing
-
-
Method Details
-
processSquare
protected boolean processSquare(GrayF32 gray, BaseDetectFiducialSquare.Result result, double edgeInside, double edgeOutside) Description copied from class:BaseDetectFiducialSquare
Processes the detected square and matches it to a known fiducial. Black border is included.- Specified by:
processSquare
in classBaseDetectFiducialSquare<T extends ImageGray<T>>
- Parameters:
gray
- Image of the undistorted squareresult
- Which target and its orientation was foundedgeInside
- Average pixel value along edge insideedgeOutside
- Average pixel value along edge outside- Returns:
- true if the square matches a known target.
-
extractNumeral
protected int extractNumeral()Extract the numerical value it encodes- Returns:
- the int value of the numeral.
-
rotateClockWise
protected void rotateClockWise() -
thresholdBinaryNumber
protected boolean thresholdBinaryNumber()Sees how many pixels were positive and negative in each square region. Then decides if they should be 0 or 1 or unknown -
findBitCounts
Converts the gray scale image into a binary number. Skip the outer 1 pixel of each inner square. These tend to be incorrectly classified due to distortion. -
setLengthSide
public void setLengthSide(double lengthSide) -
getGridWidth
public int getGridWidth()Number of elements wide the grid is -
setAmbiguityThreshold
public void setAmbiguityThreshold(double ambiguityThreshold) parameters which specifies how tolerant it is of a square being ambiguous black or white.- Parameters:
ambiguityThreshold
- 0 to 1, inclusive
-
getNumberOfDistinctFiducials
public long getNumberOfDistinctFiducials() -
getGrayNoBorder
-
getBinaryInner
-
printClassified
public void printClassified()
-